In this tutorial, we’ll solve adam’s charity question also Adam decides to be generous and do some charity programs using different languages.
This question is asked in so many competitive programming exams and also in Accenture coding round. So, here you’ll get the exact and complete solution to this question.
So let us see how this question can be solved using different programming languages with explanations.
Table of Contents
Adam’s Charity
Adam decides to be generous and do some charity. Starting today, from day 1 until day n, he gives i^2 coins to charity on day ‘i’ (1<=i<=n). Return the total coins he would give to charity.
Input Specification:
Input 1: Number of days of the Charity.
Output Specification:
Return the total number of coins till the charity days.
Example 1:
Input 1: 2
Output: 5
Explanation: There is a total of 2 days.
Example 2:
Input 1: 3
Output: 14
Explanation: There is a total of 3 days.
Now we’ll code this question using C, C++, Java, and Python.
Adam Decides to be Generous Solution Using C
//Learnprogramo - programming made simple #include <stdio.h> #include <math.h> int main() { int n,i; scanf("%d",&n); int sum = 0; for(i=1;i<=n;i++) sum += pow(i,2); printf("%d",sum); return 0; }
Output:
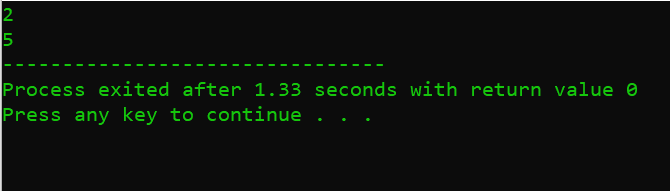
Explanation:
We’ve coded adam decides to be a generous program using C language. After compiling the above program the compiler will ask you to enter the input n i.e total no of days. Then the for loop will be executed till the condition i<=n becomes false. At last, the output is printed on the screen.
Now we’ll code the same Adam decides to be a generous program using C++ language.
Adam’s Charity Program Using C++
//Learnprogramo - programming made simple #include <iostream> #include <math.h> using namespace std; int main() { int n; cin >> n; int sum = 0; for(int i=1;i<=n;i++) sum += pow(i,2); cout << sum; return 0; }
Output:
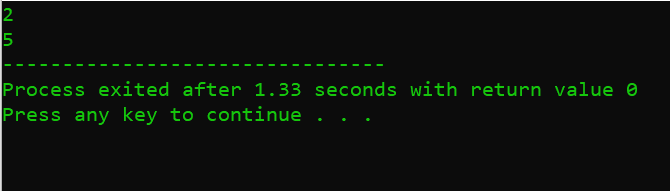
Explanation:
In the above program, we’ve used the C++ language. All the code is the same as C only the syntax is different. First, you’ve to enter the number of days then for loop gets executed till the condition i<=n get false. Then at last the total sum is printed on the screen.
Adams Charity Program Using Java
//Learnprogramo - programming made simple import java.util.*; public class Learnprogramo { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); int sum = 0; for(int i=1;i<=n;i++) sum += Math.pow(i,2); System.out.println(sum); } }
Output:
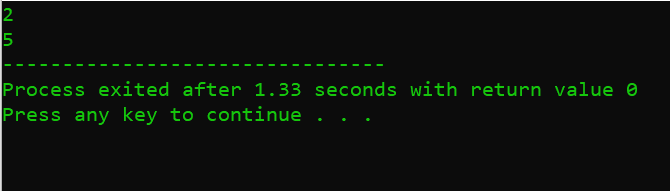
Explanation:
We’ve used the java inbuilt function of the util library Scanner to accept the no of days from the user. Also, we’ve used Math.pow function to get the power of any number.
Adam Decides to be generous in Python
#Learnprogramo - programming made simple n=int(input()) sum=0 for i in range(1,n+1): sum=sum+(i**2) print(sum)
Output:
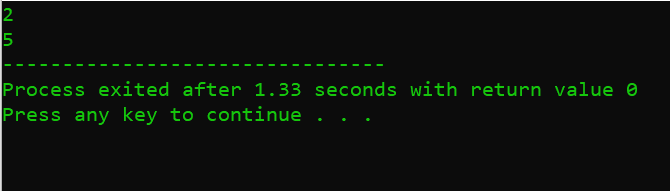
Conclusion
In this way, we’ve solved Adams Charity’s question using different programming languages. If you’ve any doubts then please feel to contact us. We’ll reach you as soon as possible.
Thanks and Happy Coding :)