Today in this lesson, we’ll see how to code NxNxN Matrix Python 3 Program and also the NxNxN Matrix python program. If you’re wondering how the NxNxN matrix program is written in python, then you’re at the right place. Here you’ll learn how to write the NxNxN matrix program using python with an explanation. But before start writing the program we’ll first learn about the NxNxN matrix. So,
Table of Contents
What is NxNxN Matrix?
The NxNxN matrix is also called as NxNxN cube or NxNxN puzzle. It is also pronounced as N by N by N. The length, height, and the width of the NxNxN matrix cube will be the same i.e same dimensions. For example: 1x1x1, 2x2x2, 3x3x3, 4x4x4 …. NxNxN matrix.
The matrix is nothing but a set of numbers that are arranged in the form of rows and columns which forms a rectangular array. The numbers of matrices are also called entries of the matrix. If the Matrix is 4×5 then the 4 represents the no of rows and the 4 represents the no of columns of that matrix.
Now we’ll see how to write python code to create the NxNxN matrix.
Create NxNxN Matrix in Python 3 with Non-Duplicating Numbers
With the help of this method, the numbers will not be repeated in rows as well as in columns. This method is widely used in the puzzles like Sudoko.
Program:
#Learnprogramo - programming made simple # Python Program to create nxn Matrix import numpy as np # Provide the value of N N = 5 # returns evenly spaced values row = np.arange(N) # create an new array filled with zeros of given shape and type result = np.zeros((N, N)) # Logic to roll array elements of given axis for i in row: result[i] = np.roll(row, i) print(result)
Output:
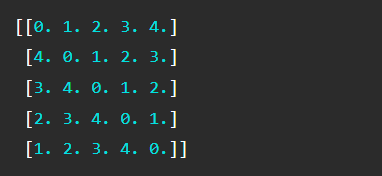
Explanation:
In this program, we’re using the python library called Numpy. First, we’ve given the size of the matrix as 5 which is stored in the variable N. Then, the line row= np.arrange(N) arranges the data and returns the evenly spaced values. result = np.zeros((N, N)) creates a new array filled with zeros of the given shape and type. At last, we’re using for loop to roll array elements of the given axis and print the matrix.
Create NxNxN matrix in Python using Numpy
In this method, we’ll create the NxNxN matrix using Python 3. You just have to change the value of N based on your requirements and the shape you needed. To generate the standard Rubik’s cube the dimensions will be 3x3x3 so, the value of n will be 3.
Program:
#Learnprogramo - programming made simple # Program to nxnxn matrix python 3 import numpy as np # Provide the value of nxnxn n = 3 a = np.arange(n) b = np.array([a]*n) matrix = np.array([b]*n) #creating an array containg n-dimensional points flat_mat = matrix.reshape((int(matrix.size/n),n)) #just a random matrix we will use as a rotation rotate = np.eye(n) + 2 #apply the rotation on each n-dimensional point result = np.array([rotate.dot(x) for x in flat_mat]) #return to original shape result=result.reshape((n,n,n)) print(result)
Output:
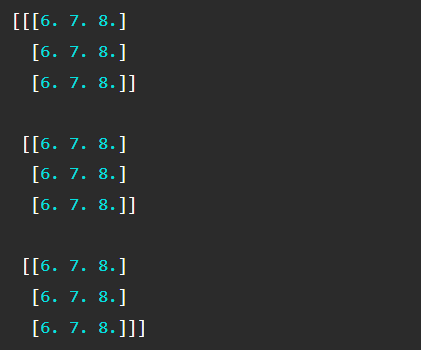
Explanation:
In this program also we’re using the Numpy python library for the creation of the NxNxN matrix. First, we’re storing the dimension of the matrix in that variable n and then creating an array containing n-dimensional points. At last, the program returns the original shape and then prints the matrix.
Create A Square Matrix in Python Program Using For Loop
There is also one method in python using which we can create a square matrix using the for a loop. Now we’ll write a python program to generate the NxNxN matrix.
Program:
#Learnprogramo - programming made simple r = int(input("Enter the number of rows:")) c = int(input("Enter the number of columns:")) #initializing the matrix matrix = [] print("Enter the entries rowwise:") for i in range(r): a =[] for j in range(c): a.append(int(input())) matrix.append(a) #printing the matrix for i in range(r): for j in range(c): print(matrix[i][j], end = " ") print()
Output:
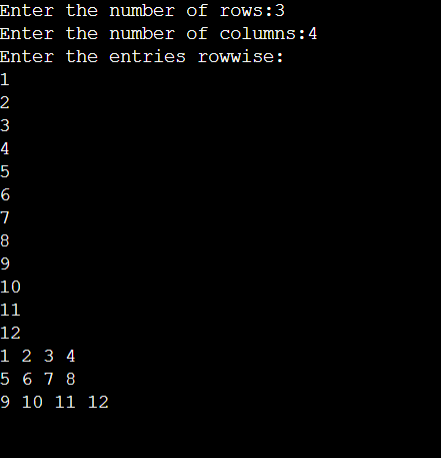
Explanation:
In this program, we’re accepting the input for rows and columns from the user. The for loop is used in this program which analyzes the range of the matrix and at last prints the sorted NxNxN matrix.
Frequently Asked Questions
Q.1 How to Create NxNxN Matrix Using Python?
Ans: We can create the NxNxN matrix in python using two methods. 1. By using the python library Numpy and 2. Using for loop to create a square matrix.
Q.2 What is NxNxN Matrix in Python?
Ans: The NxNxN matrix is also called as NxNxN cube or NxNxN puzzle. It is also pronounced as N by N by N. The length, height, and the width of the NxNxN matrix cube will be the same i.e same dimensions. For example: 1x1x1, 2x2x2, 3x3x3, 4x4x4 …. NxNxN matrix.
Conclusion
In this way, we’ve seen how to create the NxNxN Matrix Python 3 Program and how to create the NxNxN Matrix using Python. If you’ve any doubts then please feel free to contact us, we’ll resolve your doubt as soon as possible.
Thanks and Happy Coding :)
Also Read: