Today we will discuss AMCAT Coding Questions and also AMCAT Automata Coding Questions. But, before discussing the questions we will make you know about AMCAT Automata. So,
Table of Contents
What is AMCAT Automata?
After cracking the AMCAT successfully the shortlisted candidates have to give the programming exam called as AMCAT Automata. The Automata exam is a little bit simple but, needs excellent logical thinking skills.
AMCAT Automata exam is like do or die, which means if you don’t clear this exam then you will be disqualified and forget about the placement. It doesn’t matter how much you score in the other rounds.
This exam is generally based on pattern programs. So you need to practice more and more pattern programs using C, C++, and java.
AMCAT Coding Questions
There are two AMCAT Coding Questions asked in the exam. In which the first question is based on the patterns and the second question is based on the looping concept.
You have to solve these two questions using any of the programming languages from C, C++ and Java. The exam time is 45 minutes where the student should solve two questions in the given time. The exam consists of an online compiler where you have to run the given programs successfully.
According to the analysis of the students, you will get one question easy and the other quiet harder. The student who solves any of the one questions without errors the probability of selection of that student increases.
So first you start attempting the first question and then focus on the second question. To crack this exam you should be having good programming skills.
AMCAT Coding Questions Pattern
No of Questions | Difficulty Level | Cut-off |
---|---|---|
2 | 1st easy and 2nd medium. | solve 1 question completely without error or solve two questions partially. |
One of the positive sides is AMCAT Automata exam doesn’t consist of the negative marking scheme.
The first question is based on different pattern programs and the second question is based on Matrices or strings etc. The second question is harder than the first question so it is asked from the hard topic like matrices or strings.
Evaluation Of Codes
The candidate’s codes are evaluated on the basis of whether the code is of basic or advance level. The written code is industry compatible or not. The complexity of the program is also checked.
The codes are also evaluated on how many errors and warning does the compiler shown. And the time taken by the candidate to solve the questions. For this you may need English Tutoring.
AMCAT Placement Paper Analysis
According to our analysis To get Selected in AMCAT student have to suffer from four placement rounds. The first round is of Aptitude test second of the coding test, third of Technical interview and the last round is of HR interview.
Rounds | No of questions | Time |
---|---|---|
Aptitude Test | 74 | 95 Minutes |
Coding Exam | 2 | 45 Minutes |
Technical Interview | – | – |
HR Interview | – | – |
AMCAT Coding Questions
- Pattern Programs.
- Loop Based Programs.
- GCD Programs.
- Find Prime Numbers up to n.
- Remove vowel from the given String.
- Elimination of repeated letter from an array.
- Make a String Reverse.
Most Commonly Asked AMCAT Coding Questions in 2021
We have divided the question based on their difficulty.
Easy:
- Find GCD and LCM of two numbers.
- Program to check whether the given number is prime or not.
- To check whether the number is Armstrong.
- Program to check number is strong or not.
- Check whether the given number is Automorphic or not.
- To print the Fibonacci series up to the n value.
- Prime number printing within a given range.
Medium:
- Pyramid pattern printing program.
- Reversing a number.
- Printing the Armstrong numbers between the given intervals.
- Converting Binary number to Decimal and vice versa.
- Decimal to Octal conversion.
- Binary to octal conversion.
Hard:
- Program for Palindrome pattern printing.
- Removing the vowels from the string.
- Finding the frequency of the characters from the given string.
- Program to find roots of the quadratic equation.
- Diamond printing pattern programs.
- Finding the largest palindrome number from the given array.
1. Pattern Programs
C program to print pyramid star patterns:
In this program, we will ask the user how many star pattern you want. Then, the user will type the integer and the compiler will start printing the star patterns on the screen.
//Learnprogramo #include<stdio.h> int main() { int row, c, n, temp; printf("Enter the number of rows in pyramid of stars you wish to see "); scanf("%d",&n); temp = n; for ( row = 1 ; row <= n ; row++ ) { /* for spacing purpose */ for ( c = 1 ; c < temp ; c++ ) printf(" "); temp--; /* for printing stars */ for ( c = 1 ; c <= 2*row - 1 ; c++ ) printf("*"); printf("\n"); } }
Output:
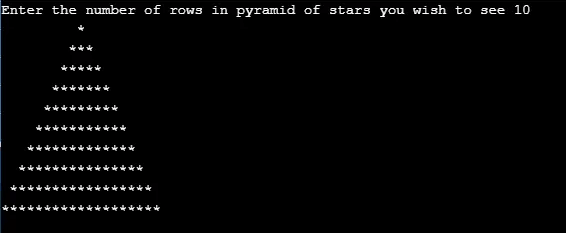
C program to print Pascal Number Triangle:
//Learnprogramo #include<stdio.h> long factorial(int); int main() { int row, c, n, temp; printf("Enter the number of rows you wish to see in pascal triangle \n"); scanf("%d",&n); temp = n; for ( row = 0 ; row < n ; row++ ) { /* for spacing purpose */ for ( c = 1 ; c < temp ; c++ ) printf(" " ); temp--; /* for printing stars */ for ( c = 0 ; c <= row ; c++ ) printf("%ld ",factorial(row)/(factorial(c)*factorial(row-c))); printf("\n"); } } long factorial(int n) { int c; long int result = 1; for (c = 1; c <= n; c++) result = result*c; return result; }
Output:
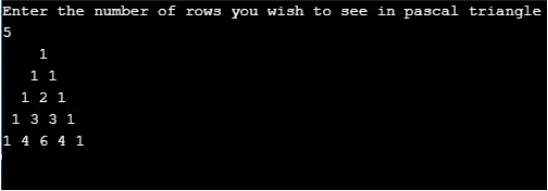
C program to print Floyd’s Triangle:
//Learnprogramo #include<stdio.h> int main() { int n, row, c, a = 1; printf("Enter the number of rows of Floyd's triangle to print\n"); scanf("%d", &n); for (row = 1; row <= n; row++) { for (c = 1; c <= row; c++) { printf("%d ",a); a++; } printf("\n"); } }
Output:
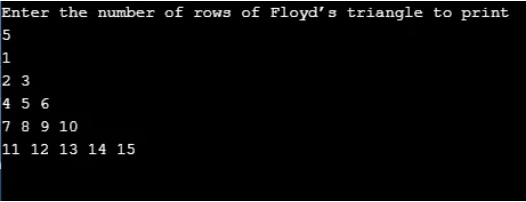
2. GCD Programs
C program to find GCD of two numbers:
//Learnprogramo #include<stdio.h> void main() { int n1,n2,i,min,GCD; printf("Enter two integers to find their GCD: \n "); scanf("%d%d",&n1,&n2); min = (n1<n2)?n1:n2; for(i=1;i<=min;i++) { // Checks if i is factor of n1 &n2 if(n1%i==0 && n2%i==0) { GCD = i; } } printf("\n G.C.D of %d and %d is %d", n1, n2, GCD); }
Output:
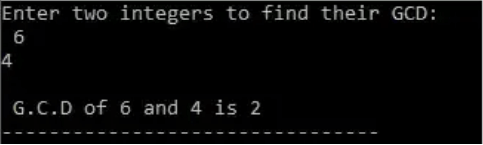
Recently Asked AMCAT Coding Questions
1st Question: Print all the prime numbers below 50 separated by the comma.
//Learnprogramo #include<stdio.h> int main() { int n,i,j,ct=0; scanf("%d",&n); for(i=2;i<=n;i++) { ct=0; for(j=2;j<i;j++) { if(i%j==0) { ct=1; break; } } if(ct==0) { if(i>2) printf(","); printf("%d",i); } } return 0; }
Output:

2nd Question: Find all the possible triplets from the array that can form the triangle.
//Learnprogramo #include<stdio.h> int arr[100], n, n1, n2, i, j, k; int a,b,c; int main() { scanf("%d",&n); for(i=0;i<n;i++) scanf("%d",&arr[i]); for(i=0;i<n-2;i++) { a=arr[i]; for(j=i+1;j<n-1;j++) { b=arr[j]; for(k=j+1;k<n;k++) { c=arr[k]; if( ((a + b)>c) && ((a + c)>b) && ((b + c)>a) ) { printf("%d %d %d",a,b,c); printf("Yes\n"); } else { printf("%d %d %d",a,b,c); printf("No\n"); } } } } return 0; }
Output:
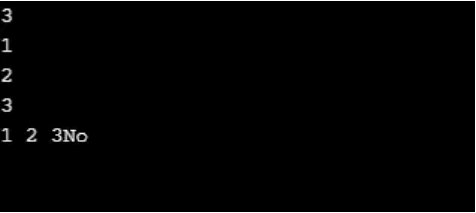
3rd Question: Write a program to find the sum of the n numbers.
//Learnrogramo #include<stdio.h> int main() { int n, i, sum = 0; printf("Enter a positive integer: "); scanf("%d", &n); for (i = 1; i <= n; ++i) { sum += i; } printf("Sum = %d", sum); return 0; }
Output:

4th Question: Program to swap two numbers.
//Learnprogramo #include<stdio.h> int main() { float A, B, temp; printf("Enter first number: "); scanf("%f", &A); printf("Enter second number: "); scanf("%f", &B); // Value of A is assigned to temp temp = A; // Value of B is assigned to A A = B; // Value of temp (initial value of A) is assigned to B B = temp; printf("\nAfter swapping, A = %.2f\n", A); printf("After swapping, B = %.2f", B); return 0; }
Output:
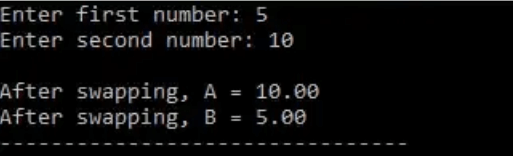
5th Question: Program to print the pattern if the input is 5.
//Learnprogramo #include<stdio.h> int main() { int i, j, k, l=1, N, d, r, count=0; scanf("%d",&N); for(i=1; i<=N; i++) { k=1; d=i%2; r=l+i-1; for(j=0;j<i;j++) { if(d==0) { printf("%d",r); r; if(k<i) { printf("*"); k=k+1; } l++; continue; } printf("%d",l); l++; if(k<i) { printf("*"); k=k+1; } } printf("\n"); } return 0; }
Output:
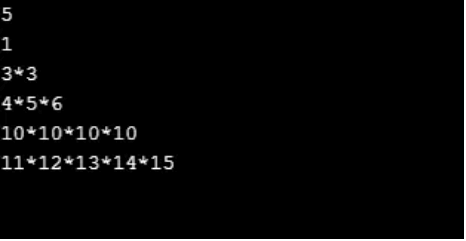
Frequently Asked Questions
1. What is AMCAT Automata Test?
The AMCAT Automata is a programming test which is conducted to evaluate the candidates who are good in programming skills.
2. What is the Syllabus of the AMCAT Automata Test?
The Syllabus for the AMCAT Automata test is programming languages like C, C++ and Java.
3. How many questions are asked in the AMCAT Automata test?
Mainly two questions are asked in the exam in which the first question is based on patterns and the second is based on strings or matrices which is hard.
4. What is Automata Pro?
The Automata pro is an exam similar to MindTree which evaluates the candidates with good programming skills.
Interview Experience
Round 1: Aptitude Test
The Aptitude Test have the following sections:
- Quantitative Ability ( 25 Questions in 35 minutes)
These sections consist of questions based on mathematics which consists of topics like probability, percentage, word and distance etc.
- English (25 Questions in 25 minutes)
Questions are based on grammar, antonyms, synonyms etc.
- Logical Ability (24 Questions in 35 minutes)
This section consists of questions based on puzzles, coding and decoding etc.
Round 2: Coding
In this section I choose C programming so the following questions that were asked are:
- Pattern Printing.
- Printing a given matrix in the spiral form.
So i attempted all the two questions but only the pattern printing question was solved without errors and the second question was partially solved.
Round 3: Technical Interview
The questions that the interviewer asked me was:
- Hi Jaydeep, How are you?
- So Jaydeep, Please introduce yourself?
- Jaydeep you are from CS background? So why you want to work as a software developer?
- Ok fine. So write a program to print the fibonacci series.
- Write a program to reverse a string.
- Program to print the elements of an array.
So, I answered all the questions. But apart from these questions interviewer also asked me questions on data structure, pointers, bubble sort, insertion sort etc. She was impressed with my answers.
So, finally, I got a call for the final HR interview.
Round 4: HR Interview
The interviewer asked me questions related to my strengths, weakness, hobbies and my family. I have answered that my hobby is to sing a song. So, the interviewer asked me a question about singing like:
- Which is your favourite Singer and why?
- Which song did you like the most of Arijit Singh?
- How many songs did Arijit Singh sung till now?
- Please sing a latest song of Arijit Singh?
I didn’t answer the third question because it was too complicated but I managed all the questions. Before leaving the room we just made a shaking hand and then I walked from the room.
After two and a half week, I got an email that congrats you have been selected for the job title software engineer. After reading this email I was totally shocked and immediately inform my all family members. They were so impressed by me.
Also Read:
- Mindtree Coding Questions
- Wipro Coding Questions
- Accenture Coding Questions
- Epam Coding Questions
- Capgemini Coding Questions
- Infosys Pseudo Code Questions