Today we’ll discuss Accenture Coding Questions and also Accenture Coding Questions with solutions, But before discussing the questions we will make you know about Accenture.
Table of Contents
What is Accenture?
Accenture is an Ireland-based multinational IT company which is specialized in the field of information technology and consulting. The Accenture comes on the list of fortune global 500 companies. Accenture was founded by Clarence DeLany in the year 1989. Its Headquarters is situated in the city of Dublin, Ireland.
Each Year Accenture conducts On-campus as well as Off-campus placements in the various parts of our country. So, in this section, we’ll see the selection criteria of Accenture and the Coding questions which are asked during the selection process of Accenture.
Accenture Coding Questions
There are 2-3 Accenture Coding Questions asked in the Exam within a time limit of 45 minutes. This is the third section of the actual test and most of our students say that the level of questions asked in the exam is of medium difficulty.
This third round is based on coding so, you’ve to practice more and more coding questions using C, C++, Java, and Python Programs. The main motive of this section is to test your coding skills as well as your problem-solving techniques.
In the exam mainly two problem statements will be given. You’ve to solve these Accenture coding questions using any of the programming languages from C, C++, Java, and python.
Highlights of Accenture Coding Section
From the year 2020 to 2021 Accenture has changed the selection process for its candidates. They’ve introduced three sections for the selection of the right candidates. The first section is Technical Assessment, the Second is Cognitive Assessment and the Third section is Coding Section. In this post, we’re going to see the third section i.e Coding Section of Accenture.
Test | Coding |
---|---|
No of questions Asked | 2 or 3 |
Difficulty Level | Medium or High |
Salary | 4.5 to 6.5 lpa |
Duration | 45 Minutes |
Section | Third |
Mainly 2 or 3 Coding questions are asked in the exam. You’ve to solve all the questions in the time period of 45 minutes. The difficulty level of these questions will be medium-high. If you want to crack the exam in the first attempt then you’ve practice more and more coding questions which are asked in the previous years.
You can solve these questions using any of the programming languages from C, C++, Java, and Python. So you don’t need to master all the programming languages, just master any of the one programming language. You can use congrapps for interview tips.
Marking Scheme of Accenture Coding Section
The candidates who clear all the first two rounds will have to go through the third round which is the coding round. All the candidates will have to solve 2 questions in 45 minutes. So to pass the third round and get your dream job at least you’ve to achieve one partial output. But the second question should be completely solved.
So to get passed in this section the candidate should get one partial output and one complete output.
Section | No of Questions | Selection Criteria |
---|---|---|
Coding Section | 2 | 1 complete and 1 partial output |
Rules for Accenture Coding Questions Section
- Two questions will be asked. You’ve to solve both the questions in 45 minutes.
- You must start your code from the scratch.
- The Coding section is divided into two, first for writing the code and the other for the output. So you should write the whole program.
- Errors are clearly displayed.
- If you want to clear this round then you must get one clear output and one partial output.
Accenture Coding Questions
Small Large Sum
Q.1 Write a function SmallLargeSum(array) which accepts the array as an argument or parameter, that performs the addition of the second largest element from the even location with the second largest element from an odd location?
Rules:
- All the array elements are unique.
- If the length of the array is 3 or less than 3, then return 0.
- If Array is empty then return zero.
Sample Test Case 1:
Input:
6
3 2 1 7 5 4
Output:
7
Explanation: The second largest element in the even locations (3, 1, 5) is 3. The second largest element in the odd locations (2, 7, 4) is 4. So the addition of 3 and 4 is 7. So the answer is 7.
Sample Test Case 2:
Input:
7
4 0 7 9 6 4 2
Output:
10
Solution: At first we’ve to take input from the user i.e in the main function. Then we’ve to create two different arrays in which the first array will contain all the even position elements and the second one will contain odd position elements. The next step is to sort both the arrays so that we’ll get the second-largest elements from both the arrays. At last, we’ve to add both the second-largest elements that we get from both the arrays. Display the desired output in the main function.
Program:
//Learnprogramo - programming made simple #include <bits/stdc++.h> using namespace std; int smallLargeSum(int *arr, int n) { if(n <= 3) { return 0; } //Here we use vector because we don't know the array size, //we can use array also but vector gives us more functionality than array vector<int> arrEven, arrOdd; //Break array into two different arrays even and odd for(int i = 0; i < n; i++) { //If Number is even then add it into even array if(i % 2 == 0) { arrEven.push_back(arr[i]); } else { arrOdd.push_back(arr[i]); } } //Sort the even array sort(arrEven.begin(), arrEven.end()); //We use sort function from C++ STL library //Sort the odd array sort(arrOdd.begin(), arrOdd.end()); //Taking second largest element from both arrays and add them return arrEven[1] + arrOdd[1]; } // Driver code int main() { int n; cout<<"Enter How many elements you want to enter?\n"; cin>>n; int arr[n]; //Get input from user cout<<"Start entering the numbers\n"; for(int i = 0; i < n; i++) { cin>>arr[i]; } cout<<"Output is\n"; cout<<smallLargeSum(arr, n); return 0; }
Output:
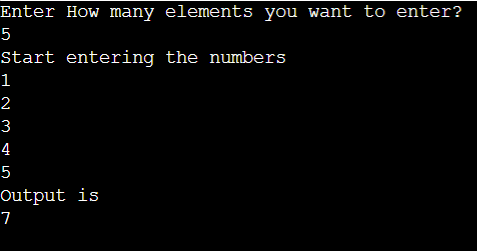
Check Password
Q.2 Write a function CheckPassword(str) which will accept the string as an argument or parameter and validates the password. It will return 1 if the conditions are satisfied else it’ll return 0?
The password is valid if it satisfies the below conditions:
- It should contain at least 4 characters.
- At least 1 numeric digit should be present.
- 1 Capital letter should be there.
- Password should not contain space or slash.
- The starting character should not be a number.
Sample Test Case:
Input:
bB1_89
Output:
1
Program:
//Learnprogramo - programming made simple #include<iostream> #include<string.h> using namespace std; int CheckPassword(char str[]) { int len = strlen(str); bool isDigit = false, isCap = false, isSlashSpace=false,isNumStart=false; //RULE 1: At least 4 characters in it if (len < 4) return 0; for(int i=0; i<len; i++) { //RULE 2: At least one numeric digit in it if(str[i]>='0' && str[i]<='9') { isDigit = true; } //RULE 3: At least one Capital letter else if(str[i]>='A'&&str[i]<='Z'){ isCap=true; } //RULE 4: Must not have space or slash if(str[i]==' '|| str[i]=='/') isSlashSpace=true; } //RULE 5: Starting character must not be a number isNumStart = (str[0]>='0' && str[0]<='9'); //FYI: In C++, if int data type function returns the true then it prints 1 and if false then it prints 0 return isDigit && isCap && !isSlashSpace && !isNumStart; } int main() { char password[100]; cout<<"Enter the Password\n"; cin>>password; cout<<"The output is =\n"; cout<<CheckPassword(password); }
Output:
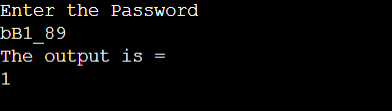
Calculate Binary Operations
Q.3 Write a function CalculateBinaryOperations(str) that accepts the string as an argument or parameter. The string should contains the binary numbers with their operators OR, AND, and XOR?
- A Means the AND Operation.
- B Means the OR Operation.
- C Means the XOR Operation.
By scanning the given string from left to right you’ve to calculate the string and by taking one operator at a time then return the desired output.
Conditions:
- The priority of the operator is not required.
- The length of the string is always Odd.
- If the length of the string is null then return -1.
Sample Test Case:
Input:
1C0C1C1A0B1
Output:
1
Explanation:
The entered input string is 1 XOR 0 XOR 1 XOR 1 AND 0 OR 1.
Now calculate the string without an operator priority and scan the string characters from left to right. Now calculate the result and return the desired output.
Note: This will convert the char into the num (char – ‘0’) in the c++ language.
Program:
//Learnprogramo - programming made simple #include <bits/stdc++.h> using namespace std; int CalculateBinaryOperations(char* str) { int len = strlen(str); //Let's consider the first element as a answer (because string can be a single char) int ans = str[0]-'0'; for(int i=1; i<len-1; i+=2) { int j=i+1; //Performing operation for AND if(str[i]=='A') { ans = ans & (str[j]-'0'); } //Performing operation for OR else if(str[i]=='B') { ans = ans | (str[j]-'0'); } //Performing operation for XOR else if(str[i]=='C') { ans = ans ^ (str[j]-'0'); } } return ans; } int main() { char str[100]; cout<<"Enter the String:\n"; cin>>str; cout<<"The output is :\n"; cout<<CalculateBinaryOperations(str); }
Output:
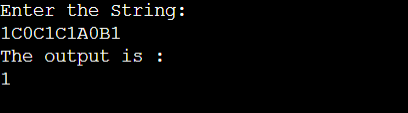
Find Maximum In An Array
Q.4 Write a function FindMaxInArray, which will find the greatest number from an array with its desired index? The greatest number and its desired index should be printed in separate lines.
Sample Test Case:
Input:
10
15 78 96 17 20 65 14 36 18 20
Output:
96
2
Program:
//Learnprogramo - programming made simple #include<iostream> using namespace std; void FindMaxInArray(int arr[],int length) { int max=-1, maxIdx=-1; for(int i = 0;i < length; i++) { if(arr[i] > max) { max = arr[i]; maxIdx = i; } } cout<<"The Maximum element in an array is = \n"; cout<<max; cout<<"\nAt the Index = \n"; cout<<maxIdx; } int main() { int n; cout<<"How many elements you want to enter:\n"; cin>>n; int a[n]; cout<<"Enter elements: \n"; for(int i=0;i<n;i++) cin>>a[i]; FindMaxInArray(a,n); }
Output:
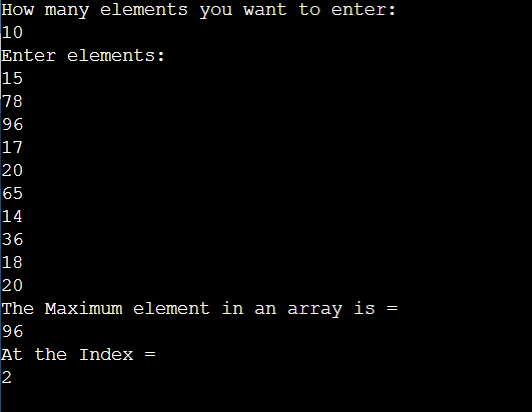
Operation Choices
Q.5 Write a function OperationChoices(c, a, b) which will accept three integers as an argument, and the function will return:
- (a + b) if the value of c=1.
- (a – b) if the value of c=2.
- (a * b) if the value of c=3.
- (a / b) if the value of c=4.
Sample Test Case:
Input:
2
15
20
Output:
-5
Here, the value of the c is two i.e 2. So it’ll perform the operation as subtraction (15, 20) and will return -5.
Program:
//Learnprogramo - programming made simple #include<iostream> using namespace std; int operationChoices(int c, int a , int b) { if(c==1) { return a + b; } else if(c==2) { return a - b; } else if(c==3) { return a * b; } else if(c==4) { return a / b; } } int main() { int x, y, z; int result; cout<<"Enter c\n"; cin>>x; cout<<"Enter two elements\n"; cin>>y; cin>>z; result = operationChoices(x, y, z); cout<<"The result is "; cout<<result; }
Output:
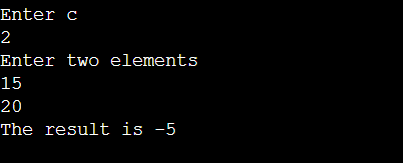
Difference Of Sum
Q.6. Write a function differenceofSum(a,b) which will take two integers as an argument. You’ve to obtain the total of all the integers ranging from 1 to n (both inclusive) that are not divisible by b. You should also return the distinction between the sum of the integers which are not divisible by b with the sum of the integers divisible by b?
Consider: a and b are greater than 0. i.e a>0 and b>0. And their sum should lies between the integral range.
Sample Test Case 1:
Input:
a = 6 and b = 30
Output:
285
Explanation:
The integers that are divisible by 6 are 6, 12, 18, 24, and 30 and their sum is 90. The integers that are not divisible by 6 are 1, 2, 3, 4, 5, 7, 8, 9, 10, 11, 13, 14, 15, 16, 17, 19, 20, 21, 22, 23, 25, 26, 27, 28 and 29. And their addition is 375.
The difference between them is (375 – 90) = 285.
Sample Test Case 2:
Input:
a = 10
b = 3
Output:
19
Anagram Strings
Q.7 Write a function to check whether the given strings are anagrams or not. If the given strings are anagram then return ‘yes’ otherwise return ‘no’?
Sample Test Case 1:
Input:
1st: learnprogramo
2nd: simple
Output:
no
Sample Test Case 2:
Input:
1st: Listen
2nd: Silent
Output:
yes
Explanation:
The two strings Listen and Silent are anagrams because rearranging all the characters from the second string forms the first string.
Product Small Pair
Q.8 Write a function Productsmallpair(sum, arr) which will accept the two integers sum and arr. These two integers will be used to find the arr(j) and arr(k) where k is not equal to j.arr(j) and arr(k). k != j.arr(j) and arr(k) should be the smallest elements from the array.
Rules:
- If the value of n<2 or empty, then return -1.
- If these pairs are not found then return the value as 0.
- You should make sure that all the values are between the range of integers.
Sample Test Case 1:
Input:
sum: 9
arr: 5 4 2 3 9 1 7
Output:
2
Solution:
From the given array of integers, you’ve to select the two smallest integers which are 1 and 2. The addition of these two numbers is (1 + 2 = 3) which is less than 9(3 < 9). And the product of these two is 2 (2 x 1 = 2) so, the output we get is 2.
Sample Test Case 2:
Input:
sum: 4
arr: 9 8 -7 3 9 3
Output:
-21
Replace Character
Q.9 Write a function Replacecharacter(Char str1, Char ch1, Int 1, Char ch2) which has a string(str) and the two characters ch1 and ch2. Execute a function in such a way that string str will return to its original string, and all the events in ch1 are replaced by ch2 and vice versa?
Consider: The strings will have only alphabets in lower case.
Sample Test Case:
Input:
str: learnprogramo
ch1: a
ch2: p
Output:
leprnarpgrpmo
Solution:
All the ‘a’s in the string are replaced with the ‘p’ and ‘p’ is replaced with the ‘a’.
Reverse a String
Q.10 Write a function that will accept strings from the user and will reverse the string word-wise. The last word will come as the first word in the output and vice versa?
Sample Test Case 1:
Input:
learn programo
Output:
programo learn
Explanation:
The reverse string word-wise function is applied.
Sample Test Case 2:
Input:
Welcome to Learnprogramo
Output:
Learnprogramo to Welcome
Frequently Asked Accenture Coding Questions
Q.1 In which programming language we should solve all the Accenture coding questions?
Ans: The students can use any of the programming languages from C, C++, Java, and Python to solve coding questions.
Q.2 Which Platform does Accenture use to conduct the exam?
Ans: Accenture uses the Cocubes platform to conduct all its exams and assessments.
Q.3 What is the difficulty level of Coding questions asked in the exam?
Ans: The first question is of medium difficulty level and the second question is very difficult.
Q.4 How to clear Accenture Coding Questions Round?
Ans: To clear the coding round of Accenture you’ve to master any of the programming languages C, C++, Java, and Python and solve maximum repeated questions asked in the previous exams.
Q.5 How many rounds are there in Accenture Selection Process?
Ans: There is a total of 3 selection rounds conducted by Accenture to get desired dream job.
Q.6 Does Accenture have any sectional cut-off?
Ans: Yes the Accenture has sectional as well as subsectional cut-offs. The candidate who clears both the cut off will be eligible for the next rounds.
Q.7 Is NSR compulsory for Accenture?
Ans: Yes, NSR is compulsory for Accenture selection document verification.
Q.8 Is it compulsory to clear Accenture Coding Round?
Ans: Yes, it’s compulsory to clear Accenture Coding Round.
Q.9 Do Accenture Coding questions get repeated each year?
Ans: Yes, our students say that they got some repeated questions which were asked in the previous exams. Mainly the questions from an inheritance, polymorphism, and constructors in C++. And from C the questions from the topic scope of variables, pointers, and structures get repeated.
Q.10 Will Accenture will hire this year?
Ans: as per the reports yes, Accenture is going to hire this year also. So start preparing for the exam.
Conclusion
So in this way we’ve solved Accenture Coding Questions with solutions. If you’ve any doubts please feel free to contact us, we’ll try to reply as soon as possible.
Thanks and Happy Coding :)
Also Read:
- Amcat Coding Questions.
- Mindtree Coding Questions.
- Cocubes Test.
- TCS Codevita.
- Zoho Aptitude Questions.
- Wipro Coding Questions.
- Hexaware Interview Questions.
- Capgemini Exam Pattern.